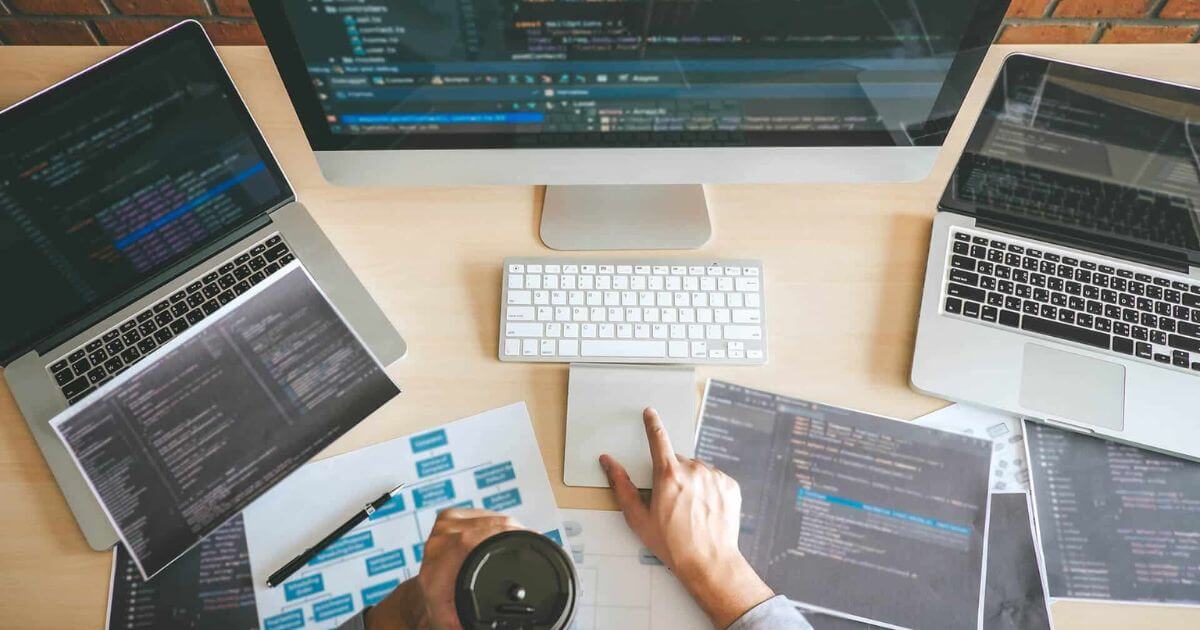
Mastering the Art of Writing Clean and Maintainable Code: Best Practices Revealed
The importance of clean and maintainable code
As a seasoned software developer, I know firsthand the importance of writing clean and maintainable code. In an industry where projects can span years and involve multiple contributors, the ability to create code that is easy to understand, modify, and scale is crucial. Clean code not only improves the efficiency of the development process but also enhances the overall quality and longevity of the software product.
When code is well-structured, self-documenting, and follows consistent naming conventions, it becomes a valuable asset that can be easily maintained and extended over time. This, in turn, reduces the risk of bugs, streamlines the debugging process, and enables faster feature development and deployment. Ultimately, investing in clean and maintainable code can save your organization time, money, and headaches down the line.
Benefits of writing clean and maintainable code
The benefits of writing clean and maintainable code are numerous and far-reaching. By prioritizing code cleanliness, you can:
-
Enhance Code Readability: Well-structured, consistently named, and adequately commented code is easier for both new and experienced developers to understand, reducing the time and effort required to onboard new team members or revisit old code.
-
Improve Code Maintainability: Clean code is more resilient to changes, making it simpler to debug, refactor, and extend functionality without introducing new bugs or breaking existing functionality.
-
Increase Developer Productivity: When code is easy to navigate and understand, developers can work more efficiently, spending less time deciphering complex logic and more time delivering new features and improvements.
-
Reduce Technical Debt: By prioritizing code cleanliness from the outset, you can minimize the accumulation of technical debt, which can often lead to costly and time-consuming refactoring efforts down the line.
-
Facilitate Collaboration: Well-documented, consistent code enables seamless collaboration among team members, fostering a shared understanding of the codebase and facilitating smoother code reviews and knowledge sharing.
-
Enhance Code Quality: Clean code is inherently more reliable, scalable, and maintainable, leading to higher-quality software products that better meet the needs of end-users.
Common challenges in writing clean and maintainable code
While the benefits of clean and maintainable code are clear, achieving this level of code quality is not without its challenges. Some of the common obstacles developers face include:
-
Tight Deadlines and Pressure to Deliver: In the fast-paced world of software development, there is often pressure to deliver new features quickly, which can lead to cutting corners and sacrificing code quality.
-
Lack of Consistent Coding Standards: Without a shared understanding of best practices and coding conventions, individual developers may adopt their own styles, resulting in an inconsistent codebase.
-
Insufficient Documentation and Knowledge Sharing: When code is not properly documented and the rationale behind design decisions is not communicated, it becomes increasingly difficult for new team members to understand and maintain the codebase.
-
Complexity and Technical Debt Accumulation: As software systems grow in complexity over time, the accumulation of technical debt can make it increasingly challenging to keep the codebase clean and maintainable.
-
Resistance to Change: Some developers may be reluctant to adopt new tools, practices, or processes that could improve code quality, preferring to stick with familiar but less effective methods.
To overcome these challenges, it is essential to establish a culture of code cleanliness and empower developers with the necessary knowledge, tools, and resources to write and maintain high-quality code.
Best practices for naming variables and functions
One of the fundamental aspects of clean and maintainable code is the way variables and functions are named. Adhering to consistent naming conventions not only enhances code readability but also helps to convey the purpose and intent of each element in the codebase.
When naming variables and functions, consider the following best practices:
-
Use Descriptive and Meaningful Names: Avoid generic or ambiguous names like
x
,data
, orprocess
. Instead, choose names that clearly describe the purpose or functionality of the variable or function, such ascustomerName
,calculateTotalRevenue
, orvalidateInputData
. -
Follow Naming Conventions: Adopt a consistent naming style, such as camelCase or snake_case, and stick to it throughout the codebase. This helps to maintain a cohesive and professional appearance.
-
Use Appropriate Abbreviations: If you need to use abbreviations, ensure they are widely recognized and consistently applied. Avoid creating your own ad-hoc abbreviations that may confuse other developers.
-
Differentiate Between Similar Concepts: When dealing with related variables or functions, use naming conventions that clearly distinguish between them. For example,
customerName
andclientName
orcalculateTotalRevenue
andcalculateNetProfit
. -
Avoid Misleading Names: Ensure that the name accurately reflects the purpose of the variable or function. Avoid names that are misleading or do not align with the actual functionality.
-
Consider the Scope and Lifetime: The naming convention may vary depending on the scope and lifetime of the variable or function. For instance, global variables may have a more descriptive name, while loop counters or temporary variables can have shorter, more concise names.
By following these best practices, you can create a codebase that is intuitive, self-documenting, and easy to maintain over time.
Structuring code for readability and maintainability
In addition to naming conventions, the overall structure and organization of your code play a crucial role in ensuring its readability and maintainability. Here are some best practices to consider:
-
Modularize and Organize Code: Break down your codebase into smaller, self-contained modules or components that encapsulate specific functionality. This makes the code easier to understand, test, and maintain.
-
Implement Separation of Concerns: Ensure that each module or component is responsible for a single, well-defined task or feature. This helps to minimize complexity and promote code reuse.
-
Use Consistent Indentation and Formatting: Adopt a consistent code formatting style, such as those prescribed by popular style guides (e.g., AirBnB, Google, or PEP 8 for Python), and apply it consistently throughout the codebase.
-
Keep Functions and Methods Concise: Aim to write functions and methods that are focused, with a single, clearly defined responsibility. Avoid creating overly long or complex functions that can be difficult to understand and maintain.
-
Leverage Code Reuse: Identify common functionality or patterns in your codebase and extract them into reusable functions, modules, or libraries. This not only promotes code cleanliness but also enhances development efficiency.
-
Implement Appropriate Error Handling: Ensure that your code gracefully handles errors and exceptions, providing clear and informative feedback to users and developers.
-
Utilize Version Control Effectively: Leverage version control systems, such as Git, to manage and track changes to your codebase. This enables collaboration, code reviews, and the ability to revert to previous working states if necessary.
By following these best practices for code structure and organization, you can create a codebase that is easy to navigate, understand, and maintain over time.
Commenting and documenting your code effectively
Effective code documentation is a crucial aspect of writing clean and maintainable code. Well-documented code not only helps current team members understand the codebase but also facilitates onboarding for new developers and enables smoother collaboration.
When it comes to commenting and documenting your code, consider the following best practices:
-
Provide Contextual Comments: Use comments to explain the purpose, functionality, and any relevant context for each module, function, or significant code block. Avoid simply restating what the code already does.
-
Explain the "Why," Not Just the "What": In addition to describing what the code does, use comments to explain the reasoning behind design decisions, the rationale for specific implementation choices, and any known limitations or trade-offs.
-
Use Consistent Formatting and Syntax: Adopt a consistent commenting style, such as the one prescribed by popular documentation tools (e.g., JavaDoc, Doxygen, or Sphinx), and apply it consistently throughout the codebase.
-
Document Public Interfaces: Ensure that all public-facing interfaces, such as API endpoints, library functions, or module exports, are thoroughly documented with clear descriptions of their purpose, parameters, return values, and any relevant usage examples.
-
Maintain Documentation Alongside Code: Treat code documentation as an integral part of the development process. Update comments and documentation as the codebase evolves to ensure they remain accurate and relevant.
-
Leverage Automated Documentation Generation: Utilize tools like JavaDoc, Doxygen, or Sphinx to automatically generate comprehensive documentation from your code comments, making it easier to maintain and publish documentation alongside your codebase.
-
Write Readme Files and Project Documentation: In addition to inline code comments, maintain high-level project documentation, such as README files, that provide an overview of the project, its architecture, and any necessary setup or usage instructions.
By following these best practices for code commenting and documentation, you can create a codebase that is not only clean and maintainable but also easily understandable and accessible to both current and future developers.
Using version control to manage and track changes
Version control systems, such as Git, are an essential tool for writing and maintaining clean and maintainable code. By leveraging version control, you can:
-
Track Changes and Revisions: Version control allows you to track changes to your codebase over time, enabling you to revert to previous working states, understand the history of a file or feature, and identify the source of any issues or bugs.
-
Facilitate Collaboration: Version control systems enable seamless collaboration among team members, allowing multiple developers to work on the same codebase simultaneously and merge their changes efficiently.
-
Implement Code Reviews: Version control systems, coupled with code review tools, enable you to establish a code review process, where team members can provide feedback, suggest improvements, and ensure code quality before merging changes.
-
Maintain a Clean and Linear Git History: Adopt best practices for Git commit messages, branching strategies, and merge workflows to keep your Git history clean, organized, and easy to navigate.
-
Leverage Branching and Merging Strategies: Use feature branches, hotfix branches, and other branching strategies to isolate changes, minimize the risk of conflicts, and maintain a clear and understandable Git history.
-
Integrate with Continuous Integration and Deployment: Integrate your version control system with Continuous Integration (CI) and Continuous Deployment (CD) tools to automate the build, test, and deployment processes, ensuring that your codebase remains clean and stable.
-
Enable Efficient Collaboration and Knowledge Sharing: Version control systems, along with tools like code review platforms, enable seamless collaboration, code reviews, and knowledge sharing among team members, fostering a culture of code cleanliness and maintainability.
By incorporating version control best practices into your development workflow, you can create a robust and reliable system for managing and tracking changes to your codebase, ultimately leading to a more maintainable and high-quality software product.
Testing and debugging techniques for clean code
Effective testing and debugging practices are essential for writing and maintaining clean and maintainable code. By incorporating these techniques into your development process, you can:
-
Implement Comprehensive Unit Tests: Write unit tests that cover the core functionality of your codebase, ensuring that individual components work as expected and reducing the risk of introducing new bugs during refactoring or feature additions.
-
Utilize Integration and End-to-End Testing: Implement integration and end-to-end tests to validate the correct functioning of your application as a whole, verifying that different components work together seamlessly.
-
Automate Testing Processes: Leverage Continuous Integration (CI) tools to automate the running of your test suite, ensuring that all changes are thoroughly tested before being merged into the codebase.
-
Adopt Test-Driven Development (TDD): Consider following a TDD approach, where you write tests before implementing the actual functionality. This helps to ensure that your code is designed with testability in mind, leading to a more modular and maintainable codebase.
-
Employ Effective Debugging Techniques: When issues or bugs arise, use a systematic approach to debugging, leveraging tools like debuggers, logging, and tracing to identify the root cause of the problem and implement a robust solution.
-
Analyze and Address Technical Debt: Regularly review your codebase and identify areas where technical debt has accumulated. Prioritize refactoring and cleanup efforts to maintain a clean and maintainable codebase over time.
-
Encourage a Culture of Testing and Code Quality: Foster a culture within your team that values testing, code quality, and continuous improvement. Provide training and resources to help developers hone their testing and debugging skills.
By incorporating these testing and debugging best practices into your development workflow, you can ensure that your codebase remains clean, reliable, and maintainable over time, reducing the risk of introducing new bugs and enabling faster and more efficient feature development.
Continuous integration and delivery for maintaining code quality
Continuous Integration (CI) and Continuous Delivery (CD) are essential practices for maintaining code quality and ensuring the long-term maintainability of your codebase. By implementing CI/CD, you can:
-
Automate Build and Test Processes: Leverage CI tools to automatically build, test, and validate your codebase with every commit or merge, catching issues early in the development cycle.
-
Enforce Code Quality Standards: Integrate static code analysis tools into your CI/CD pipeline to automatically check for code style, best practices, and potential issues, ensuring that your codebase adheres to predefined quality standards.
-
Streamline Deployment and Release Processes: CD pipelines enable you to automate the deployment of your application to various environments, reducing the risk of manual errors and ensuring consistent and reliable releases.
-
Implement Continuous Monitoring and Feedback: Integrate monitoring and observability tools into your CI/CD pipeline to track the performance, stability, and user experience of your application, allowing you to quickly identify and address any issues that arise.
-
Foster a Culture of Collaboration and Transparency: CI/CD practices promote a culture of collaboration, where team members can easily see the status of the codebase, the progress of ongoing work, and the impact of their changes.
-
Enable Faster Iterations and Feedback Loops: By automating the build, test, and deployment processes, CI/CD allows you to iterate more quickly, get faster feedback, and make informed decisions about the codebase.
-
Facilitate Rollbacks and Disaster Recovery: CI/CD pipelines provide the ability to quickly roll back to a previous stable version of your application, enabling you to recover from issues or bugs without significant downtime.
By implementing a robust CI/CD pipeline, you can ensure that your codebase remains clean, maintainable, and of high quality throughout the entire software development lifecycle.